Integrating TensorFlow into Your Machine Learning Projects
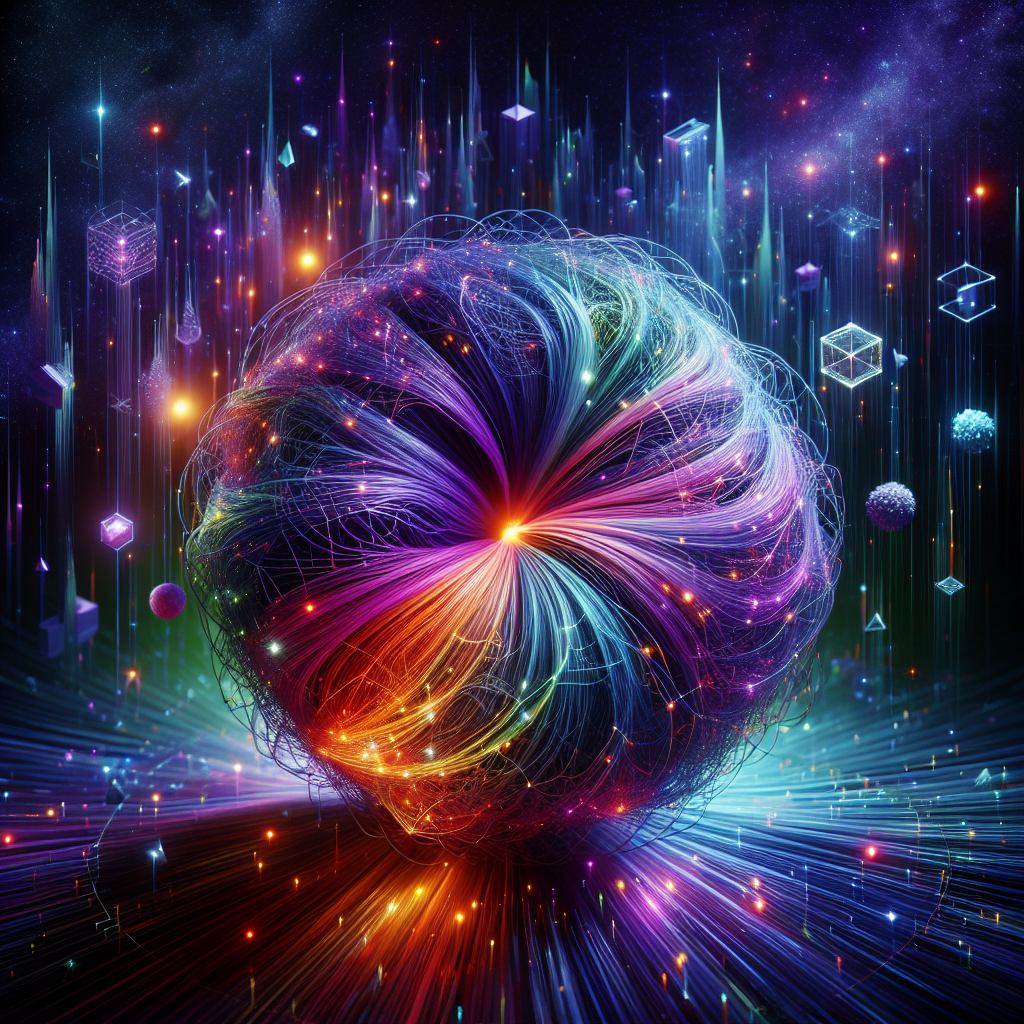
Integrating TensorFlow into Your Machine Learning Projects
Machine learning has transformed how we interact with data, providing insights that were once elusive. At the forefront of this revolution is TensorFlow, a powerful open-source machine learning framework developed by Google. Known for its versatility in developing neural networks and other deep learning models, TensorFlow can significantly enhance your machine learning projects’ capabilities. This comprehensive guide will walk you through integrating TensorFlow into your projects with practical tutorials, setup guides, and valuable insights.
Introduction
As the demand for sophisticated data analysis grows, so does the need for robust tools that simplify these tasks. TensorFlow, developed by Google, stands out as one of the leading machine learning frameworks in this space. Whether you’re tackling image recognition, natural language processing, or predictive analytics, TensorFlow provides a comprehensive ecosystem to support your projects. This post will explore how integrating TensorFlow into your machine learning workflows can elevate your capabilities and streamline deep learning model implementation.
Setting Up TensorFlow
Prerequisites
Before diving into TensorFlow, ensure your system meets the following prerequisites:
- Python: TensorFlow supports Python 3.5–3.8.
- Anaconda or Miniconda (optional): These are useful for managing environments.
- GPU Support (optional but recommended for deep learning tasks): NVIDIA CUDA and cuDNN libraries.
Installation Guide
Install via pip: The easiest method is through Python’s package manager:
pip install tensorflow
Installation with Anaconda:
Create a new environment:
conda create --name tf_env python=3.8
Activate the environment and install TensorFlow:
conda activate tf_env conda install tensorflow
For GPU support, follow TensorFlow’s official guide to set up NVIDIA drivers, CUDA Toolkit, and cuDNN.
Leveraging TensorFlow in Machine Learning Projects
Understanding the Core Components
TensorFlow is built around key components that facilitate seamless model development:
- Tensors: The fundamental data structure, representing multidimensional arrays.
- Graphs: These define computations by specifying how tensors interact with each other.
- Sessions: Manage the execution of graphs and provide methods to evaluate nodes or compute gradients.
Integrating TensorFlow can significantly enhance the capabilities of your machine learning projects. TensorFlow is widely used in developing neural networks and other deep learning models, making it a crucial tool for modern Machine Learning Projects.
TensorFlow Tutorials for Projects
To get started with TensorFlow tutorials for projects, begin by exploring basic operations such as tensor manipulations, matrix multiplications, and gradient computations. These foundational elements will help you understand the framework’s capabilities and prepare you for more complex model implementations.
Example: Basic Tensor Operations
import tensorflow as tf
# Define a constant tensor
a = tf.constant([[1, 2], [3, 4]])
b = tf.constant([[5, 6], [7, 8]])
# Perform element-wise addition
result_add = tf.add(a, b)
print("Addition result:\n", result_add.numpy())
# Matrix multiplication
result_matmul = tf.matmul(a, b)
print("Matrix multiplication result:\n", result_matmul.numpy())
This example demonstrates how to perform basic tensor operations in TensorFlow, which are crucial for building and training neural networks.
Building Your First Neural Network
Let’s dive into a simple example of creating a neural network using TensorFlow’s Keras API. This is often the first step many practitioners take when integrating TensorFlow into their machine learning projects.
Example: Simple Neural Network with Keras
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense
# Define a simple sequential model
model = Sequential([
Dense(10, activation='relu', input_shape=(784,)),
Dense(10)
])
# Compile the model
model.compile(optimizer='adam',
loss=tf.losses.SparseCategoricalCrossentropy(from_logits=True),
metrics=['accuracy'])
print("Model built successfully!")
This simple neural network can be used for tasks like digit classification on datasets such as MNIST. It’s a great starting point for beginners to understand how models are constructed in TensorFlow.
Advanced Features and Best Practices
Using TensorFlow Datasets (TFDS)
TensorFlow provides tf.data
, an API that helps manage large datasets efficiently. Coupled with TFDS, you can easily import and preprocess data for your projects.
Example: Loading MNIST Dataset
import tensorflow_datasets as tfds
# Load the dataset
mnist_dataset = tfds.load('mnist', split='train', shuffle_files=True)
for example in mnist_dataset.take(1):
image, label = example['image'], example['label']
print("Image shape:", image.shape)
print("Label:", label.numpy())
This code loads and processes the MNIST dataset using TensorFlow Datasets, which simplifies data handling in machine learning projects.
Model Optimization Techniques
Optimizing your model can significantly enhance performance. Here are some strategies:
- Batch Normalization: Improves training speed and stability.
- Regularization: Helps prevent overfitting by adding penalties on layer parameters or activity.
- Learning Rate Scheduling: Adjusts the learning rate during training to improve convergence.
These techniques are essential for refining your machine learning models, especially when dealing with complex datasets.
Real-world Applications
TensorFlow is widely used in various domains. Here are some notable applications:
- Image Recognition: Used by companies like Google and NVIDIA to enhance computer vision systems.
- Natural Language Processing (NLP): Powers tools for translation, sentiment analysis, and more.
- Healthcare: Assists in analyzing medical images and predicting patient outcomes.
These examples illustrate how TensorFlow is used to develop sophisticated solutions across industries, showcasing its versatility and power as a machine learning framework.
Conclusion
Integrating TensorFlow into your Machine Learning Projects can significantly enhance their capabilities. With its comprehensive ecosystem and flexible architecture, it’s an ideal choice for both researchers and practitioners. From building simple neural networks to optimizing complex models, TensorFlow provides the tools necessary to transform data challenges into actionable insights.
Frequently Asked Questions
1. What makes TensorFlow different from other machine learning frameworks?
TensorFlow stands out due to its flexibility, scalability, and comprehensive ecosystem. Developed by Google, it supports a wide range of applications and is optimized for both research and production environments.
2. Is TensorFlow suitable for beginners in machine learning?
Yes, TensorFlow’s user-friendly API and extensive documentation make it accessible for beginners. Keras integration further simplifies model creation with intuitive functions and classes.
3. Can I use TensorFlow on my mobile device?
Absolutely! TensorFlow Lite is designed for deploying models on mobile and embedded devices, providing lightweight alternatives that maintain high performance.
4. How does TensorFlow handle large datasets efficiently?
TensorFlow’s tf.data
API optimizes data loading and preprocessing by enabling parallel processing, prefetching, and caching, ensuring efficient handling of large datasets.
5. What are some common use cases for TensorFlow in machine learning projects?
Common applications include image recognition, natural language processing, predictive analytics, speech recognition, and more. Its versatility makes it suitable for various domains and industries.
By integrating TensorFlow into your Machine Learning Projects and utilizing its rich features and best practices, you can transform complex data challenges into actionable insights, driving innovation across industries.