Building Virtual Assistants with PyTorch – A Beginner’s Guide
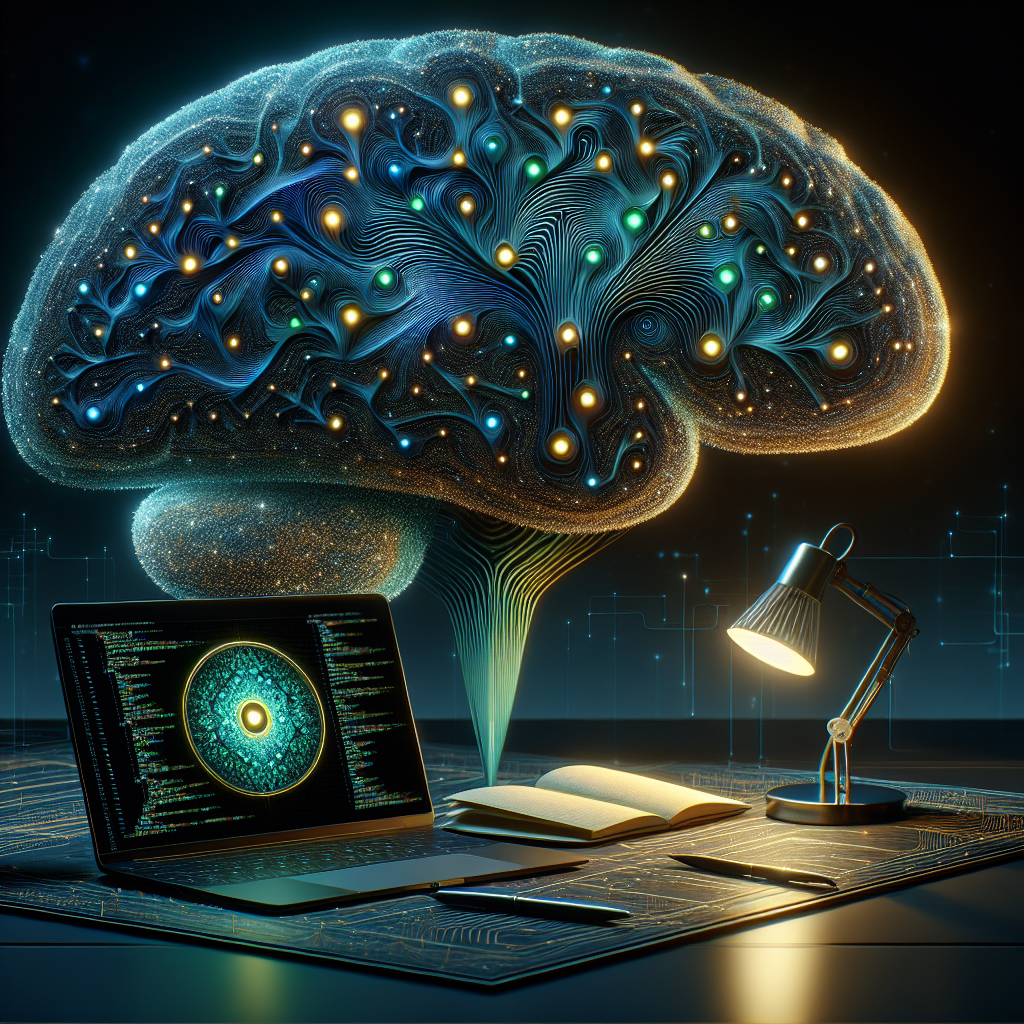
Building Virtual Assistants with PyTorch – A Guide for Beginners
Welcome to the exciting world of virtual assistant development! Whether you’re aiming to create a simple AI chatbot or an advanced smart assistant, understanding how to leverage PyTorch—a popular machine learning library developed by Facebook—can be your first step toward success. This guide will walk you through the essentials of building AI-powered assistants using PyTorch, with a focus on natural language processing (NLP) and neural networks.
Introduction
In recent years, virtual assistants have become integral parts of our digital lives, helping us manage tasks, provide information, and even entertain us. Behind these sophisticated systems lie complex algorithms powered by machine learning technologies like PyTorch. If you’re new to virtual assistant development, this guide will introduce you to the basics of neural networks in PyTorch, how to implement conversational agents using PyTorch, and more.
Why Choose PyTorch for Virtual Assistant Development?
PyTorch is celebrated for its dynamic computation graph, which allows developers to modify graphs on-the-fly. This flexibility makes it an excellent choice for building AI chatbots and smart assistants. Moreover, PyTorch’s extensive library of pre-trained models and tools simplifies the development process, making it accessible even to beginners in machine learning.
PyTorch is particularly advantageous because of its ease of use in prototyping and experimentation. Its dynamic nature allows developers to iterate quickly, which is essential when developing complex systems like virtual assistants that require fine-tuning and adjustments based on user feedback.
Additionally, PyTorch has a vibrant community and extensive documentation, making it easier for beginners to find resources, tutorials, and support as they embark on their projects. This ecosystem supports various components necessary for building sophisticated virtual assistants, including modules for deep learning and natural language processing (NLP).
Understanding the Basics of Neural Networks in PyTorch
Before diving into virtual assistant development, let’s explore the fundamental concepts of neural networks using PyTorch. These networks are at the heart of many AI systems, enabling them to learn from data and make intelligent decisions.
What Are Neural Networks?
Neural networks are computational models inspired by the human brain’s structure. They consist of layers of interconnected nodes (or “neurons”) that process input data and generate outputs. In PyTorch, these networks can be easily defined using its intuitive API.
Getting Started with PyTorch for Beginners
To begin working with neural networks in PyTorch, it’s essential to understand the core components:
- Tensors: The fundamental data structure used by PyTorch is similar to arrays and matrices.
- Autograd: This module provides automatic differentiation for all operations on Tensors. It simplifies gradient calculations, which are crucial during training neural networks.
- nn.Module: A class in PyTorch that serves as a base for building neural network layers.
Here’s an example of defining a simple feedforward neural network in PyTorch:
import torch
import torch.nn as nn
class SimpleNet(nn.Module):
def __init__(self, input_size, hidden_size, output_size):
super(SimpleNet, self).__init__()
self.hidden = nn.Linear(input_size, hidden_size)
self.relu = nn.ReLU()
self.output = nn.Linear(hidden_size, output_size)
def forward(self, x):
x = self.hidden(x)
x = self.relu(x)
x = self.output(x)
return x
# Example usage
net = SimpleNet(10, 5, 2)
print(net)
In this example, SimpleNet
consists of an input layer, a hidden layer with ReLU activation, and an output layer. This structure can be expanded to build more complex models suitable for various tasks in virtual assistant development.
Training Neural Networks
Once your neural network is defined, training it involves feeding data through the network (forward pass), calculating loss using a criterion like cross-entropy, and updating weights using backpropagation (backward pass). PyTorch simplifies this process with optimizers such as torch.optim.SGD
or torch.optim.Adam
.
import torch.optim as optim
criterion = nn.CrossEntropyLoss()
optimizer = optim.Adam(net.parameters(), lr=0.001)
# Example training loop
for epoch in range(num_epochs):
for inputs, labels in data_loader:
optimizer.zero_grad()
outputs = net(inputs)
loss = criterion(outputs, labels)
loss.backward()
optimizer.step()
Training is an iterative process where you repeatedly adjust the network’s parameters to minimize loss and improve accuracy on tasks like natural language understanding.
Implementing Conversational Agents Using PyTorch
A key component of virtual assistants is their ability to engage in conversation. This involves NLP techniques that allow the assistant to understand user input and generate appropriate responses.
Building a Simple Chatbot with PyTorch
To illustrate, let’s create a simple chatbot using sequence-to-sequence (Seq2Seq) models. These models are well-suited for tasks like machine translation and conversational agents because they can map sequences of words in one language to sequences in another.
Components of Seq2Seq Models
- Encoder: This part of the model processes the input sentence, encoding it into a fixed-size context vector.
- Decoder: It uses the context vector to generate an output sequence (response).
Here’s how you can implement a basic Seq2Seq architecture in PyTorch:
class EncoderRNN(nn.Module):
def __init__(self, input_size, hidden_size):
super(EncoderRNN, self).__init__()
self.hidden_size = hidden_size
self.embedding = nn.Embedding(input_size, hidden_size)
self.gru = nn.GRU(hidden_size, hidden_size)
def forward(self, input, hidden):
embedded = self.embedding(input).view(1, 1, -1)
output, hidden = self.gru(embedded, hidden)
return output, hidden
class DecoderRNN(nn.Module):
def __init__(self, hidden_size, output_size):
super(DecoderRNN, self).__init__()
self.hidden_size = hidden_size
self.embedding = nn.Embedding(output_size, hidden_size)
self.gru = nn.GRU(hidden_size, hidden_size)
self.out = nn.Linear(hidden_size, output_size)
self.softmax = nn.LogSoftmax(dim=1)
def forward(self, input, hidden):
embedded = self.embedding(input).view(1, 1, -1)
output, hidden = self.gru(embedded, hidden)
output = self.softmax(self.out(output[0]))
return output, hidden
# Example instantiation
encoder = EncoderRNN(input_size=10, hidden_size=256)
decoder = DecoderRNN(hidden_size=256, output_size=10)
Training the Seq2Seq Model
Training a Seq2Seq model involves passing input sequences through the encoder to generate context vectors and then using these vectors in the decoder to produce target sequences. The training process is similar to other neural networks: compute loss and adjust weights.
encoder_optimizer = optim.Adam(encoder.parameters(), lr=0.01)
decoder_optimizer = optim.Adam(decoder.parameters(), lr=0.01)
def train(input_tensor, target_tensor, encoder, decoder):
encoder_hidden = encoder.initHidden()
encoder_optimizer.zero_grad()
decoder_optimizer.zero_grad()
input_length = input_tensor.size(0)
target_length = target_tensor.size(0)
loss = 0
for ei in range(input_length):
encoder_output, encoder_hidden = encoder(input_tensor[ei], encoder_hidden)
decoder_input = torch.tensor([[SOS_token]])
decoder_hidden = encoder_hidden
use_teacher_forcing = True if random.random() < teacher_forcing_ratio else False
if use_teacher_forcing:
for di in range(target_length):
decoder_output, decoder_hidden = decoder(decoder_input, decoder_hidden)
loss += criterion(decoder_output, target_tensor[di])
decoder_input = target_tensor[di]
else:
for di in range(target_length):
decoder_output, decoder_hidden = decoder(decoder_input, decoder_hidden)
topv, topi = decoder_output.topk(1)
decoder_input = topi.squeeze().detach()
loss += criterion(decoder_output, target_tensor[di])
if decoder_input.item() == EOS_token:
break
loss.backward()
encoder_optimizer.step()
decoder_optimizer.step()
return loss.item() / target_length
Improving Conversational Abilities with Attention Mechanisms
To enhance the performance of Seq2Seq models, especially for longer sentences, attention mechanisms can be integrated. Attention allows the model to focus on different parts of the input sequence when generating each word in the output sequence.
Implementing an Attention Layer
Here’s a simple way to add an attention mechanism using PyTorch:
class AttnDecoderRNN(nn.Module):
def __init__(self, hidden_size, output_size, dropout_p=0.1, max_length=MAX_LENGTH):
super(AttnDecoderRNN, self).__init__()
self.hidden_size = hidden_size
self.output_size = output_size
self.dropout_p = dropout_p
self.max_length = max_length
self.embedding = nn.Embedding(self.output_size, self.hidden_size)
self.attn = nn.Linear(self.hidden_size * 2, self.max_length)
self.attn_combine = nn.Linear(self.hidden_size * 2, self.hidden_size)
self.dropout = nn.Dropout(self.dropout_p)
self.gru = nn.GRU(self.hidden_size, self.hidden_size)
self.out = nn.Linear(self.hidden_size, self.output_size)
def forward(self, input, hidden, encoder_outputs):
embedded = self.embedding(input).view(1, 1, -1)
embedded = self.dropout(embedded)
attn_weights = F.softmax(
self.attn(torch.cat((embedded[0], hidden[0]), 1)), dim=1)
attn_applied = torch.bmm(attn_weights.unsqueeze(0),
encoder_outputs.unsqueeze(0))
output = torch.cat((embedded[0], attn_applied[0]), 1)
output = self.attn_combine(output).unsqueeze(0)
output = F.relu(output)
output, hidden = self.gru(output, hidden)
output = F.log_softmax(self.out(output[0]), dim=1)
return output, hidden, attn_weights
# Example instantiation
decoder_with_attention = AttnDecoderRNN(hidden_size=256, output_size=10)
In this example, the AttnDecoderRNN
class uses attention to combine encoder outputs with current decoder inputs. The model calculates attention weights and applies them to focus on relevant parts of the input sequence.
Integrating Virtual Assistants with IoT Devices
Virtual assistants can be enhanced by integrating them with Internet of Things (IoT) devices, enabling more interactive and useful applications like smart home control or health monitoring.
Connecting a Virtual Assistant with Smart Home Devices
Here’s how you can connect a virtual assistant to control IoT-enabled lights using Python:
import requests
def turn_on_light(ip_address):
url = f'http://{ip_address}/api/v1.0/device/1/action'
data = {'on': True}
response = requests.post(url, json=data)
return response.status_code == 200
# Example usage
if __name__ == '__main__':
light_ip = '192.168.1.100' # Replace with your smart device IP address
if turn_on_light(light_ip):
print("Light turned on successfully!")
else:
print("Failed to turn on the light.")
This simple script sends an HTTP request to a smart light’s API, turning it on. Virtual assistants can trigger such scripts based on voice commands like “Turn on the lights.”
Handling IoT Data for Health Monitoring
Virtual assistants can also play a crucial role in health monitoring by collecting data from IoT devices:
import json
import paho.mqtt.client as mqtt
def on_connect(client, userdata, flags, rc):
print(f"Connected with result code {rc}")
client.subscribe("health/temperature")
def on_message(client, userdata, msg):
temperature = float(msg.payload.decode())
if temperature > 37.5:
notify_user('High temperature detected!')
def notify_user(message):
# This function would contain the logic to send a notification to the user
print(f"Notification: {message}")
client = mqtt.Client()
client.on_connect = on_connect
client.on_message = on_message
client.connect("mqtt.example.com", 1883, 60) # Connect to your MQTT broker
client.loop_forever() # Start listening for messages
This example uses the MQTT protocol to subscribe to temperature data from a health monitoring device. If a high temperature is detected, it triggers a notification.
Conclusion
Virtual assistants are evolving into powerful tools that can not only manage tasks through voice commands but also interact with physical devices in our environment. By integrating them with IoT technologies and enhancing their conversational abilities with advanced NLP techniques like attention mechanisms, we’re paving the way for smarter, more intuitive interactions between humans and machines.
FAQs
Q: What are virtual assistants?
A: Virtual assistants are AI-powered software agents that can perform tasks or services based on commands. They use natural language processing to understand voice commands and are integrated into various applications and devices.
Q: Can virtual assistants be used in healthcare?
A: Yes, virtual assistants can be used for health monitoring by integrating with IoT devices like fitness trackers or smart thermometers. They can track health metrics and alert users or healthcare providers about potential health issues.
Q: How do attention mechanisms improve conversational abilities?
A: Attention mechanisms allow a model to focus on different parts of the input sequence when generating each word in the output, improving its ability to handle long sentences and context-specific references. This results in more accurate and relevant responses from virtual assistants.
Q: What is MQTT, and how does it relate to IoT?
A: MQTT (Message Queuing Telemetry Transport) is a lightweight messaging protocol designed for small sensors and mobile devices. It’s widely used in IoT applications for device-to-device communication due to its low power usage and efficient bandwidth consumption.