Deep Learning with PyTorch – Best Practices
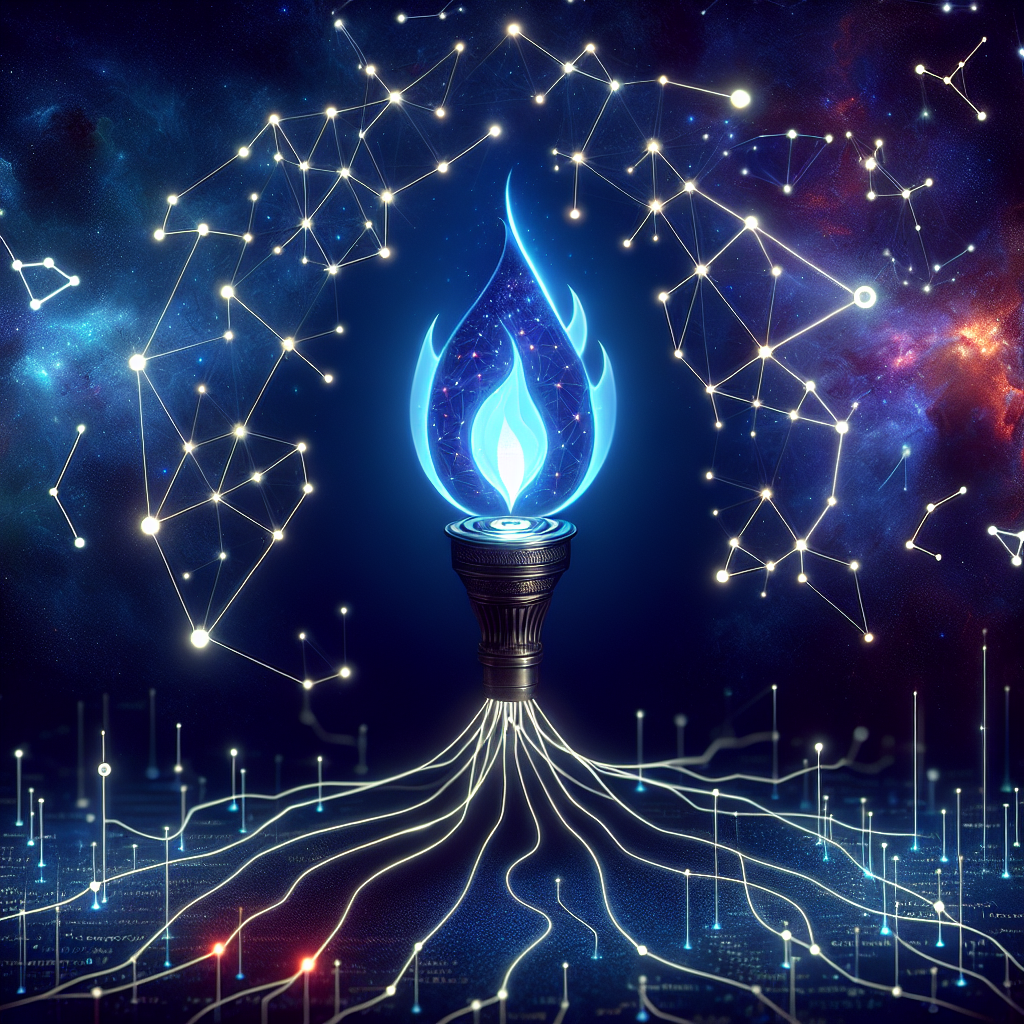
In an era where artificial intelligence (AI) is driving unprecedented innovation across industries, deep learning models have emerged as key players in reshaping business landscapes. These powerful tools enable companies to analyze vast amounts of data and gain insights that drive strategic decision-making. However, many organizations face challenges when attempting to harness these complex models effectively. The root cause often lies in inefficient training loops, suboptimal model architectures, and inadequate data preprocessing—all contributing to extended development cycles and compromised results.
The consequences of such inefficiencies are significant: they lead to increased computational costs, delayed product launches, and missed opportunities in competitive markets. For business professionals seeking a strategic advantage through AI, mastering best practices for deep learning with PyTorch can be transformative.
The Rise of Deep Learning
Deep learning, a subset of machine learning, leverages neural networks with multiple layers (hence “deep”) to model complex patterns in data. Its applications range from image and speech recognition to natural language processing and autonomous systems. PyTorch, developed by Facebook AI Research (FAIR), has become one of the leading frameworks for implementing these models due to its flexibility and ease of use.
Exploring the Challenges
Understanding Causes of Inefficiency
Inefficient Training Loops: Often overlooked, inefficient training loops can lead to prolonged training times and excessive resource consumption. This inefficiency arises from not leveraging available hardware effectively or failing to optimize data handling processes.
Suboptimal Model Architectures: Without proper understanding and tuning, models may underperform or overfit, failing to deliver desired results. Selecting the right architecture for a given task is critical but often underestimated.
Neglected Data Augmentation and Preprocessing: The foundation of any deep learning project is data. Poor practices in preprocessing and augmentation can severely limit model effectiveness, as models rely on high-quality input to learn effectively.
Addressing Common Misconceptions
One Size Fits All Models: Many assume a single architecture works for all tasks, overlooking the necessity for customization. Each problem domain may require tailored approaches to achieve optimal results.
Data Quality Over Quantity: Acquiring more data without ensuring its quality and relevance often leads to disappointing outcomes. High-quality data is essential for training robust models.
A Solution Framework: Best Practices in PyTorch
To navigate these challenges effectively, we present a structured solution framework with practical steps:
Optimizing Training Loops: Efficient training loops are key to reducing both time and resource usage.
Mastering Model Architecture and Hyperparameter Tuning: Deep understanding of these aspects can significantly boost model performance.
Implementing Robust Data Augmentation and Preprocessing: Essential steps to fully leverage your dataset’s potential.
Industry Trends and Future Predictions
As AI continues to evolve, the importance of efficient deep learning practices is only set to increase. Organizations that adopt these best practices will likely lead in innovation and maintain a competitive edge. Furthermore, advancements such as automated machine learning (AutoML) tools are simplifying model selection and hyperparameter tuning, making it easier for businesses to deploy AI solutions effectively.
Implementation Guide: Step-by-Step
1. Implementing Efficient Training Loops
Step 1: Utilize DataLoader
Use PyTorch’s DataLoader
class for efficient data handling, which helps streamline the training process by batching and shuffling data seamlessly.
Example:
from torch.utils.data import DataLoader
train_loader = DataLoader(train_dataset, batch_size=64, shuffle=True)
Step 2: Leverage GPU Acceleration
Ensure your model and data are on a GPU to significantly speed up the training process. This step is crucial for handling complex models efficiently.
Example:
device = torch.device('cuda' if torch.cuda.is_available() else 'cpu')
model.to(device)
Step 3: Apply Gradient Accumulation
When dealing with large batch sizes and limited memory, gradient accumulation can be a lifesaver. This technique allows you to simulate larger batches by accumulating gradients over several smaller ones.
Example:
accumulation_steps = 4
optimizer.zero_grad()
for i, (inputs, labels) in enumerate(train_loader):
inputs, labels = inputs.to(device), labels.to(device)
outputs = model(inputs)
loss = criterion(outputs, labels)
loss.backward()
if (i + 1) % accumulation_steps == 0:
optimizer.step()
optimizer.zero_grad()
2. Understanding Model Architecture and Hyperparameter Tuning
Step 1: Choose the Right Model
Select models that are pre-trained or designed for specific tasks to save time and improve performance. Libraries like Hugging Face Transformers offer excellent resources for natural language processing (NLP) projects.
Example:
from transformers import BertModel
model = BertModel.from_pretrained('bert-base-uncased')
Step 2: Hyperparameter Tuning
Experiment with learning rates, batch sizes, and other parameters to find the best configuration for your model. Tools like Ray Tune or Optuna can automate this process, making it more efficient.
Additional Insights on Model Architecture
Understanding the nuances of various neural network architectures is essential for selecting the right one for your task. Convolutional Neural Networks (CNNs) excel in image-related tasks, while Recurrent Neural Networks (RNNs) are preferred for sequence data like text or time series. Transformers have revolutionized NLP by efficiently handling long-range dependencies.
3. Data Augmentation and Preprocessing
Step 1: Apply Data Augmentation Techniques
Enhance the diversity of your dataset with techniques such as rotation or flipping. Libraries like Albumentations offer a range of options for image data augmentation.
Example:
from albumentations import Compose, RandomCrop, HorizontalFlip
transform = Compose([RandomCrop(32, 32), HorizontalFlip()])
Step 2: Preprocess Data
Normalize and standardize your data to improve model performance. Proper preprocessing ensures that the input data is in an optimal format for training.
Example:
from torchvision import transforms
preprocess = transforms.Compose([
transforms.ToTensor(),
transforms.Normalize(mean=[0.485, 0.456, 0.406], std=[0.229, 0.224, 0.225])
])
Additional Context on Data Preprocessing
Data preprocessing involves cleaning and transforming raw data into a format suitable for model training. This may include handling missing values, encoding categorical variables, scaling numerical features, or converting text into embeddings. These steps are crucial as they directly impact the quality of input data, which in turn influences model performance.
Case Study: Successful Implementation
Consider a leading e-commerce company that improved their recommendation system by adopting these best practices:
Efficient Training Loops: By utilizing DataLoader and GPU acceleration, they reduced training time by 40%.
Optimized Model Architecture: Implementing models from Hugging Face Transformers enhanced accuracy significantly.
Enhanced Data Management: Through robust preprocessing techniques, they achieved a more reliable recommendation engine.
Additional Case Study Insights
Another example is a healthcare provider that leveraged PyTorch to develop an AI model for predicting patient outcomes. By optimizing their training loops and applying data augmentation, they reduced prediction errors by 30%, leading to better resource allocation and improved patient care.
Frequently Asked Questions
What is PyTorch?
PyTorch is an open-source machine learning library developed by Facebook AI Research (FAIR). Known for its flexibility and ease of use, it’s widely adopted in both research and production environments.
Why choose PyTorch over other libraries?
PyTorch’s dynamic computation graphs make it easier to debug and experiment with models. Its seamless integration with Python and strong community support further enhance its appeal.
How does data augmentation improve model performance?
Data augmentation increases dataset diversity by applying random transformations like rotation or flipping, helping models generalize better across varied inputs.
Can PyTorch be used for both research and production?
Absolutely! PyTorch’s flexibility allows it to serve as a powerful tool in both experimentation and deployment. Tools like TorchServe facilitate its use in production environments.
What are common pitfalls when tuning hyperparameters?
Common mistakes include overfitting due to excessive epochs or selecting inappropriate learning rates that either cause divergence or slow convergence.
Ready to Transform Your Business with AI?
Understanding the complexities of implementing deep learning solutions is crucial, which is why our AI Agentic software development and AI Cloud Agents services can be your key differentiator. We have successfully helped companies in retail, healthcare, and finance adopt cutting-edge AI technologies that drive growth and innovation.
By partnering with us, you’ll gain expert insights on optimizing training loops, mastering model architectures, and enhancing data management—mirroring the best practices outlined above. Don’t miss this opportunity; contact us for a consultation today through our easy-to-use form on this page. We’re eager to address any questions and assist in implementing these transformative AI strategies tailored to your business needs.
Embrace the future with confidence by leveraging deep learning best practices using PyTorch, and let us guide you every step of the way! With the rapid pace of technological advancements, staying ahead means continually refining your approach and embracing new tools that enhance efficiency and innovation.